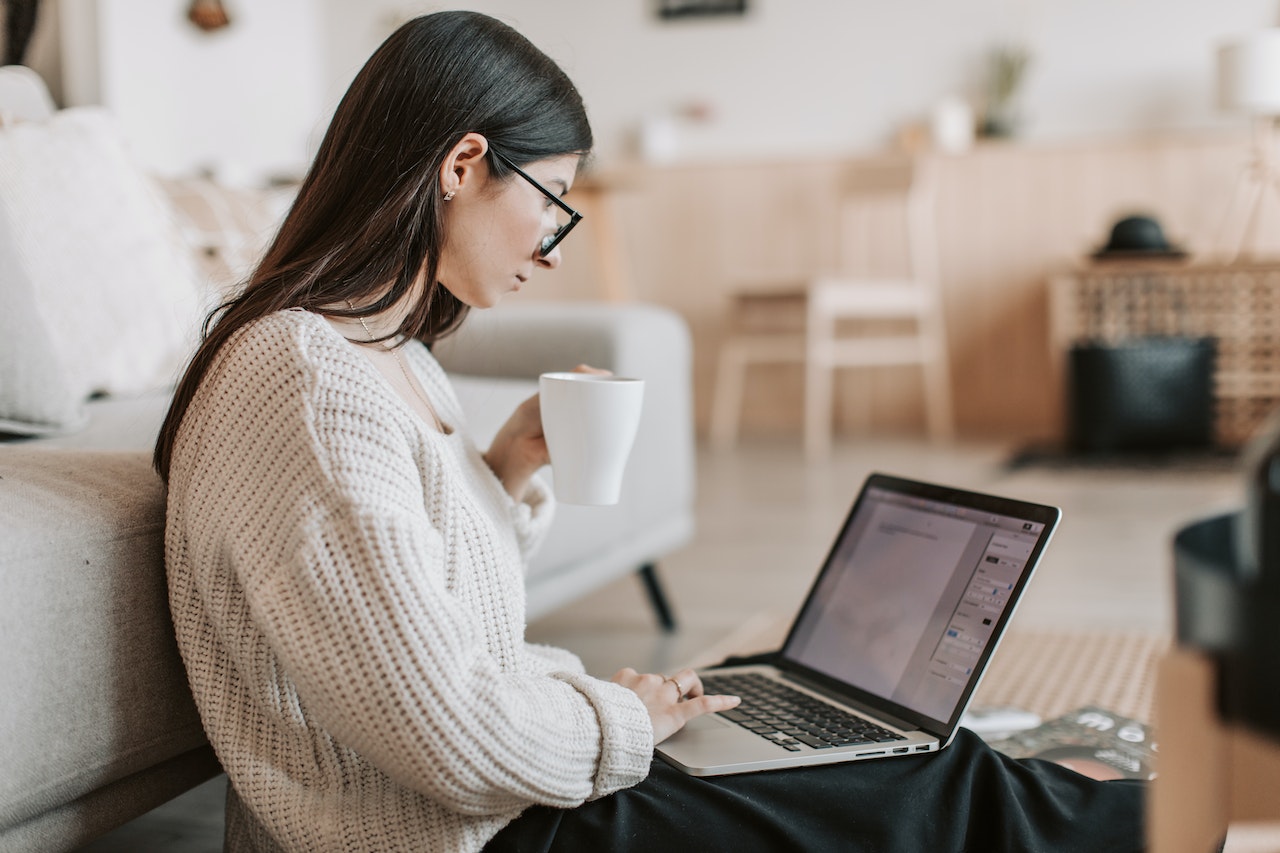
Factorial in PHP
In this article, we’ll create a program for Factorial in PHP.
Table of Contents
Factorial, a fundamental mathematical concept, is the product of an integer and all the positive integers below it.
While it may seem simple, its applications extend far beyond the realm of mathematics. In the world of programming, factorials are widely utilized in various domains, from data analysis to algorithms.
We will explore the intriguing world of factorials and unveil the power of implementing factorial in PHP, a versatile and widely adopted scripting language.
Understanding Factorial:
Before we dive into the PHP implementation, let’s take a moment to grasp the essence of factorials. The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n. Mathematically, it can be represented as:
n! = n * (n-1) * (n-2) * … * 3 * 2 * 1
The factorial of a number n is defined by the product of all the digits from 1 to n (including 1 and n).
In mathematics, the factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n. For example, 5 ! = 5 × 4 × 3 × 2 × 1 = 120
For example,
- 4! = 4*3*2*1 = 24
- 6! = 6*5*4*3*2*1 = 720
Note:
- It is denoted by n! and is calculated only for positive integers.
- Factorial of 0 is always 1.
The simplest way to find the factorial of a number is by using a loop. There are two ways to find factorial in PHP:
- Using loop
- Using recursive method
Logic:
- Take a number.
- Take the descending positive integers.
- Multiply them.
Program For Factorial in PHP
PHP, with its simplicity and flexibility, provides an ideal environment for implementing factorial calculations. Let’s explore two approaches to compute factorials in PHP:
Iterative Approach: The iterative approach involves using a loop to iteratively multiply the numbers from 1 to n. Here’s an example of a PHP function that computes the factorial using an iterative approach:
function factorialIterative($n)
{
$result = 1;
for ($i = 1; $i <= $n; $i++)
{
$result *= $i;
}
return $result;
}
// Example usage:
echo factorialIterative(5); // Output: 120
Recursive Approach: The recursive approach, as the name suggests, employs a function that calls itself to solve smaller subproblems. Here’s an example of a PHP function that calculates the factorial in php using a recursive approach:
function factorialRecursive($n)
{
if ($n == 0 || $n == 1)
{
return 1;
}
return $n * factorialRecursive($n - 1);
}
// Example usage:
echo factorialRecursive(5); // Output: 120
Both approaches yield the same result, but the choice between them depends on the specific requirements of your program and the efficiency needed.
The below program shows a form through which you can calculate the factorial in php of any number.
<html> <head> <title>Factorial Program using loop in PHP</title> </head> <body> <form method="post"> Enter the Number:<br> <input type="number" name="number" id="number"> <input type="submit" name="submit" value="Submit" /> </form> <?php if($_POST){ $fact = 1; //getting value from input text box 'number' $number = $_POST['number']; echo "Factorial of $number:<br><br>"; //start loop for ($i = 1; $i <= $number; $i++){ $fact = $fact * $i; } echo $fact . "<br>"; } ?> </body> </html>
In mathematics, factorial from original number is the results from multiplication between the numbers round positive less than or the same with n. Factorial written sebagai n! And called n factorial. In general can be written as:
n!=n.(n-1).(n-2).(n-3)…
For n that very large, it would be too exhausting to calculate n! Use both the definition. If the precision is not too important, approach from n! Can be calculated using Stirling:
n!=√2πn
Factorials play a crucial role in various programming scenarios, from permutation and combination calculations to complex algorithms like dynamic programming.
We explored the concept of factorial in php and learned how to harness the power of PHP to calculate factorials using both iterative and recursive approaches.
By implementing factorial calculations in PHP, you can unleash the potential of this versatile language and leverage factorials to solve a wide range of problems. So go ahead, experiment with factorial in PHP projects, and unlock new possibilities in the world of programming!
I hope this article helps you to create a factorial in PHP.