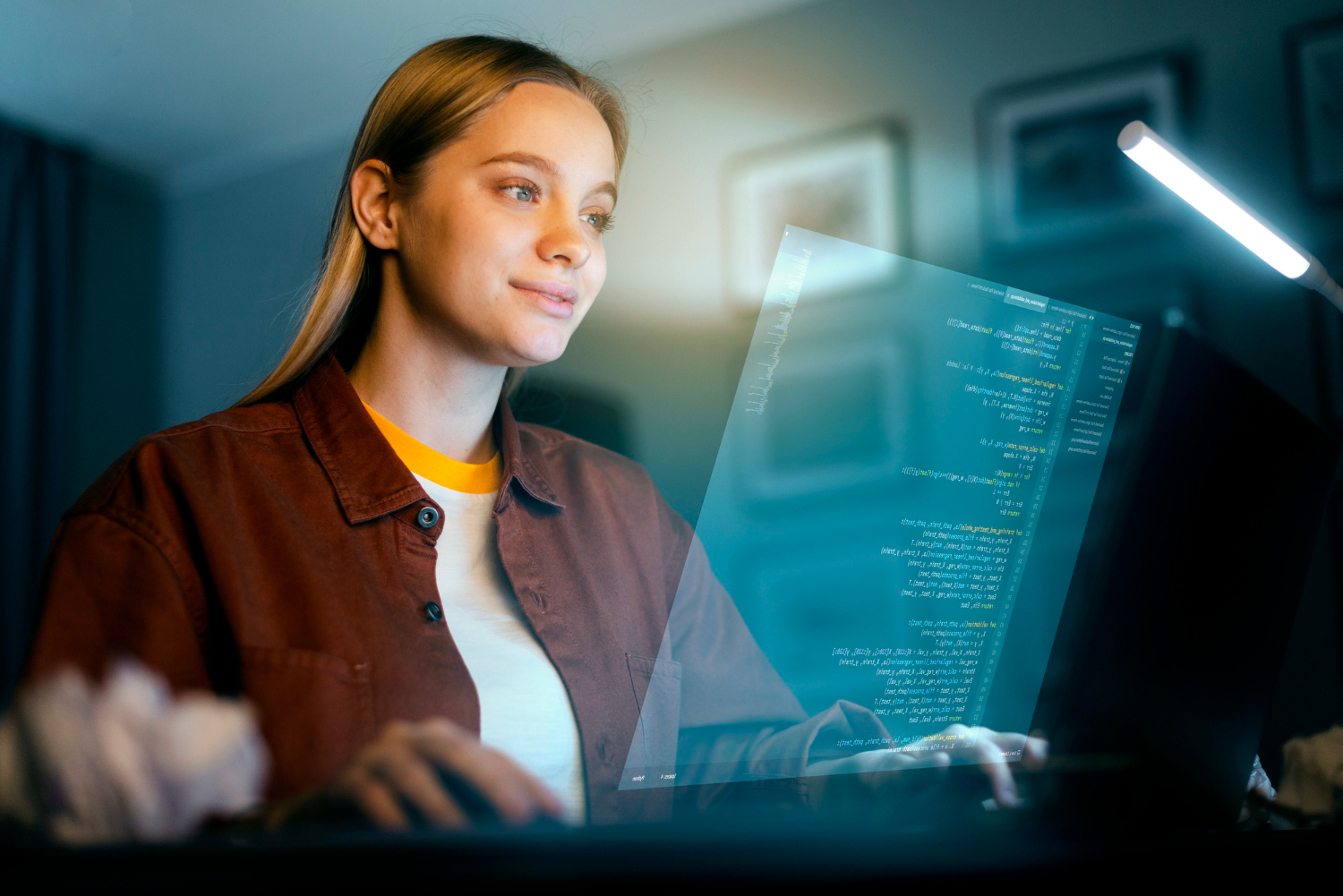
Mastering JavaScript String Methods: A Comprehensive Guide
JavaScript is a dynamic scripting language. It powers the interactive components of millions of websites. It has a powerful set of methods that can be used to manipulate strings efficiently.
Table of Contents
Strings are sequences of characters. They are an essential part of web development. Therefore, understanding JavaScript String Methods is very important for web developers.
Let’s take a look at some of the powerful and lesser-known string methods that you can use to simplify your coding and improve the performance of your web apps.
List of Javascript String Methods:
The following are list of useful Javascript String Methods to manipulate strings.
charAt() Method:
Often overlooked, the charAt()
method allows developers to retrieve the character at a specific index within a string. It’s a simple yet effective way to access individual characters and perform operations based on their values.
let str = "JavaScript"; let firstChar = str.charAt(0); // Returns 'J'
slice() Method:
The slice()
javascript string methods is a versatile tool for extracting portions of a string. It takes two parameters, the start and end indices, allowing you to create substrings with ease.
let str = "Web Development"; let substring = str.slice(0, 3); // Returns 'Web'
startsWith() and endsWith() Methods:
Simplifying conditional checks, the startsWith()
and endsWith()
methods verify whether a string begins or ends with a specific substring, respectively
let str = "Hello, World!"; let startsWithHello = str.startsWith("Hello"); // Returns true let endsWithWorld = str.endsWith("World"); // Returns false
replace() Method:
The replace()
method facilitates the replacement of a specified substring with another. This is particularly useful for dynamically updating content in response to user interactions.
let message = "Hello, Guest!"; let updatedMessage = message.replace("Guest", "User"); // Returns 'Hello, User!'
toLowerCase() and toUpperCase() Methods:
These methods are handy for normalizing string casing. Whether you need to make user inputs case-insensitive or convert text to uppercase for display purposes, toLowerCase()
and toUpperCase()
have got you covered.
let mixedCase = "RaNdom CasE"; let lowerCase = mixedCase.toLowerCase(); // Returns 'random case' let upperCase = mixedCase.toUpperCase(); // Returns 'RANDOM CASE'
split() Method:
The split()
method breaks a string into an array of substrings based on a specified delimiter. This is particularly useful when dealing with data in a delimited format, such as CSV or tab-separated values.
let csvData = "John,Doe,30,Developer"; let dataArray = csvData.split(","); // Returns ['John', 'Doe', '30', 'Developer']
indexOf() and lastIndexOf() Methods:
The indexOf()
javascript string methods return the index of the first occurrence of a specified substring within a string. Conversely, the lastIndexOf()
method returns the index of the last occurrence.
let sentence = "The quick brown fox jumps over the lazy dog."; let indexOfFox = sentence.indexOf("fox"); // Returns 16 let lastIndexOfDog = sentence.lastIndexOf("dog"); // Returns 44
trim() Method:
The trim()
javascript string methods remove leading and trailing whitespaces from a string, which is especially useful when handling user inputs to ensure data integrity.
let userInput = " Hello, World! "; let trimmedInput = userInput.trim(); // Returns 'Hello, World!'
concat() Method:
The concat()
javascript string methods combine two or more strings, creating a new string without modifying the existing ones. This is an alternative to the +
operator for string concatenation.
let firstName = "John"; let lastName = "Doe"; let fullName = firstName.concat(" ", lastName); // Returns 'John Doe'
match() Method:
The match()
javascript string methods are used with regular expressions to search for matches within a string. It returns an array containing all matched substrings.
let text = "The code is 123-4567."; let matches = text.match(/\d+/g); // Returns ['123', '4567']
repeat() Method:
The repeat()
javascript string methods create a new string by repeating the original string a specified number of times. This can be especially handy when generating repetitive patterns or building strings dynamically.
let pattern = "abc"; let repeatedPattern = pattern.repeat(3); // Returns 'abcabcabc'
localeCompare() Method:
The localeCompare()
method compares two strings in the current locale and returns a numerical value indicating their relative order. This is useful for sorting strings alphabetically with consideration for language-specific rules.
let string1 = "apple"; let string2 = "banana"; let comparisonResult = string1.localeCompare(string2); // Returns a negative value since 'apple' comes before 'banana'
padStart() and padEnd() Methods:
The padStart()
and padEnd()
javascript string methods pad a string with a specified character until it reaches a specified length. This is particularly helpful for formatting strings to a certain width.
let number = "42"; let paddedNumber = number.padStart(5, '0'); // Returns '00042'
substring() Method:
Similar to the slice()
method, the substring()
method extracts characters from a string between two specified indices. However, unlike slice()
, substring()
cannot accept negative indices.
let str = "JavaScript is amazing!"; let subStr = str.substring(0, 10); // Returns 'JavaScript'
String.fromCharCode() and String.fromCodePoint() Methods:
These methods provide the reverse functionality of charCodeAt()
by generating a string from Unicode character codes or code points, respectively.
let charCode = 65; // Unicode for 'A' let fromCharCode = String.fromCharCode(charCode); // Returns 'A' let codePoint = 128515; // Unicode for 😃 let fromCodePoint = String.fromCodePoint(codePoint); // Returns '😃'
String.raw() Method:
The String.raw()
method returns a raw string representation of a template literal, ignoring escape characters. This can be particularly useful when working with regular expressions or any scenario where you want to preserve backslashes.
let path = String.raw`C:\Users\Username\Documents`; // Returns 'C:\\Users\\Username\\Documents'
String.prototype.trimStart() and String.prototype.trimEnd() Methods:
These javascript string methods are similar to trim()
, but they specifically trim whitespaces from the beginning (trimStart()
) or end (trimEnd()
) of a string.
let spacedString = " Trim me! "; let trimmedStart = spacedString.trimStart(); // Returns 'Trim me! ' let trimmedEnd = spacedString.trimEnd(); // Returns ' Trim me!'
String.prototype.includes() Method:
The includes()
method checks whether a string contains a specific substring and returns a boolean value. It’s a concise way to perform simple existence checks.
let sentence = "The quick brown fox jumps over the lazy dog."; let containsFox = sentence.includes("fox"); // Returns true
String.prototype.search() Method:
The search()
method searches a string for a specified value or regular expression. It returns the index of the first match or -1 if no match is found.
let text = "Learn JavaScript to unleash creativity!"; let indexOfJS = text.search(/JavaScript/i); // Returns 6
Credits:
- Image by Freepik
Conclusion:
In conclusion, JavaScript String Methods provide a powerful set of methods to manipulate and work with text in the web development world. By learning these methods, web developers can improve the performance and user experiences of their applications.
As you learn more about JavaScript, these string methods will become an essential part of your coding vocabulary. They will help you streamline your code and make your apps more dynamic.
So, let’s unleash the power of JavaScript String Methods on your next web development journey!