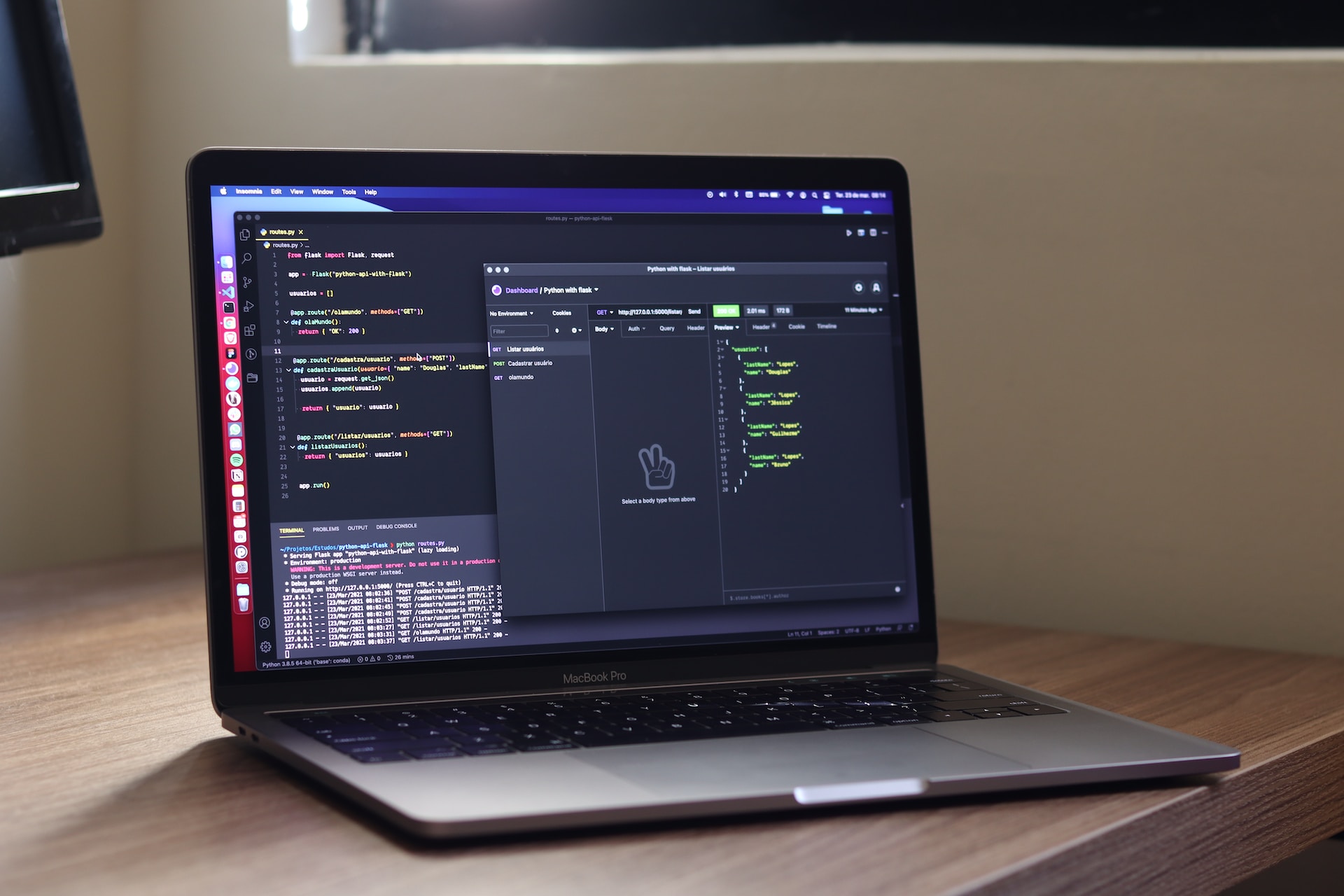
4 Useful Methods to call API in JavaScript
In this article, we’ll see about Useful Methods to call API in JavaScript
Table of Contents
Introduction
Web applications frequently rely on data from multiple sources to produce dynamic and current content in today’s linked environment. JavaScript developers frequently utilize Application Programming Interfaces (APIs) to fetch data from external sources.
You may access and modify data from servers, databases, and other web services using these potent tools. We’ll look at four key methods to call API in JavaScript.
List of methods to call API in Javascript
We’ll learn about the following 4 Methods with an example of call API in Javascript
- XMLHttpRequest
- Fetch
- Using axios library
- jQuery Ajax
1). XMLHttpRequest (XHR)
XMLHttpRequest (XHR) is a JavaScript object that provides a way to make HTTP requests to fetch data from a web server or send data to a server. It’s a fundamental part of AJAX (Asynchronous JavaScript and XML) and is commonly used for making call API in JavaScript.
It provides the foundation for AJAX (Asynchronous JavaScript and XML) requests. However, it’s important to note that XHR can be somewhat verbose and less user-friendly than newer methods, such as the Fetch API. Here’s a basic example:
var xhr = new XMLHttpRequest(); xhr.open("GET", "https://jsonplaceholder.typicode.com/posts/1", true); xhr.onreadystatechange = function () { if (xhr.readyState === 4 && xhr.status === 200) { // The API request is complete, and the response is available. var responseData = JSON.parse(xhr.responseText); console.log(responseData); } }; xhr.onerror = function () { console.error("An error occurred while making the API request."); }; xhr.send();
In this example, we use the XMLHttpRequest object to make a GET request to the JSONPlaceholder API to retrieve information about a specific post. When the request is successful, the data is parsed as JSON, and the response is logged to the console. If an error occurs during the request, the error handler logs an error message.
2). Fetch API
The Fetch API is a modern JavaScript API for making network requests (e.g., fetching data from an API) in a more user-friendly and flexible way than the older XMLHttpRequest (XHR) method.
It’s built on Promises, making working with asynchronous code and handling responses easier. The Fetch API is widely supported in modern browsers, and it’s considered a more modern and recommended approach for making call API in JavaScript.
Here’s a Fetch example:
fetch("https://jsonplaceholder.typicode.com/posts/1") .then(response => { if (!response.ok) { // Handle non-successful response (e.g., 404 Not Found, 500 Internal Server Error) throw new Error(`HTTP error! Status: ${response.status}`); } return response.json(); // Parse the response as JSON }) .then(data => { console.log(data); // Process the JSON data }) .catch(error => { console.error("Fetch API Error:", error); // Handle any errors });
In this example:
- We use
fetch()
to make a GET request to the specified URL. - The first
.then()
block checks the response status (e.g., 404, 500) and throws an error if the response is not OK. If the response is OK, it proceeds to parse the response as JSON. - The second
.then()
block handles the parsed JSON data and logs it to the console. - The
.catch()
block is used to handle any errors that occur during the request, such as network issues or server errors.
The Fetch API offers a clean and concise way to call API in JavaScript, and it’s well-suited for both simple and more complex scenarios. It simplifies the process of making asynchronous requests and handling responses and errors.
3). Axios
Axios is a popular JavaScript library that simplifies making HTTP requests to APIs in a clean and user-friendly manner.
It works both in the browser and Node.js, offering a more convenient and powerful way to interact with APIs. To use Axios, you need to include it in your project:
First, you need to install Axios. You can do this using npm or yarn:
npm install axios # or yarn add axios
In your JavaScript code, import Axios:
const axios = require("axios"); // for Node.js // or import axios from "axios"; // for modern browsers (using ES6 modules)
Here’s a practical example of making a GET request to a hypothetical API and handling the response using Axios:
const axios = require("axios"); // Import Axios axios .get("https://jsonplaceholder.typicode.com/posts/1") .then(response => { console.log(response.data); // Process the response data }) .catch(error => { console.error("Axios Error:", error); // Handle any errors });
In this example:
- We import Axios.
- We use
axios.get()
to make a GET request to the specified URL. - The first
.then()
block handles the response data, which is automatically parsed as JSON by Axios. - The
.catch()
block is used to handle any errors that occur during the request, such as network issues or server errors.
Axios is a powerful and widely used library for call API in JavaScript, and it’s particularly well-suited for applications that require easy handling of both successful responses and errors. It provides a more modern and convenient way to work with network requests compared to older methods like XMLHttpRequest (XHR).
4). jQuery Ajax
jQuery provides a convenient method called $.ajax()
for making asynchronous HTTP requests, often used to fetch data from APIs or send data to a server. The $.ajax()
method is part of the jQuery library, making it easy to use for many web developers.
For those working with legacy codebases or simply preferring jQuery, the jQuery.ajax method is a reliable option. It’s a bit more user-friendly than XHR and still gets the job done. Here’s an explanation and an example of how to use jQuery’s Ajax method:
First, make sure to include the jQuery library in your project. You can include it by adding the following script tag to your HTML:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Here’s a practical example of making a GET request to a hypothetical API and handling the response using jQuery’s Ajax method:
$.ajax({ url: "https://jsonplaceholder.typicode.com/posts/1", // API endpoint URL type: "GET", // HTTP method (GET in this case) dataType: "json", // Expected data type (JSON) success: function (data) { console.log(data); // Process the response data }, error: function (xhr, status, error) { console.error("jQuery Ajax Error:", error); // Handle any errors } });
In this example:
- We make use of
$.ajax()
to configure the API request. We specify the URL, the HTTP method (GET), and the expected data type (JSON). - The
success
callback function handles the response data, and the data is automatically parsed as JSON. - The
error
callback function is used to handle errors. If the request fails, it logs an error message.
jQuery Ajax is a powerful and user-friendly way to call API in JavaScript. It simplifies the process of making asynchronous requests, parsing JSON responses, and handling errors. While it’s widely used, note that modern JavaScript options like the Fetch API and libraries like Axios are becoming more popular due to their simplicity and better support for Promises and async/await.
Credits:
- Photo by Douglas Lopes on Unsplash
Conclusion
To call API in JavaScript is a basic web development skill. Knowing how to call API in Javascript is crucial, regardless of whether you’re using Axios, the more recent Fetch API, the classic XMLHttpRequest, or other libraries.
Additionally, by enhancing user experience and efficiency, cutting-edge methods like WebSocket, GraphQL, and async/await can assist you in elevating your apps. Keep abreast of the most recent techniques and select the one that best meets the needs of your project.
These methods will give you the tools you need to manage data retrieval and develop dynamic, interactive online applications.
I hope this article has helped to learn about Useful Methods to call API in JavaScript