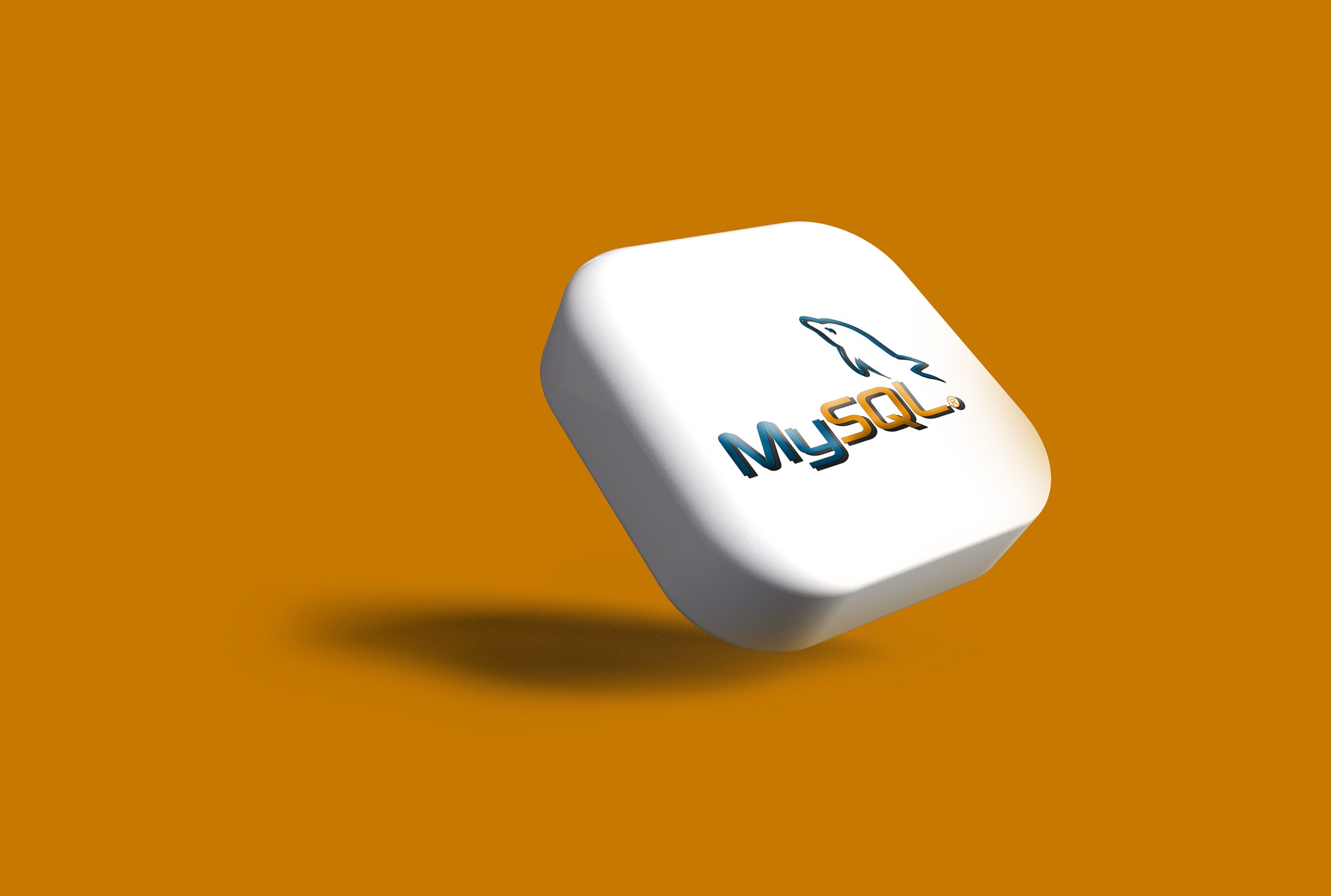
MySQL Constraints : Easy Guide to Ensuring Data Integrity
In this article, we’ll learn about MySQL Constraints, including their types, functions, and real-world implementation and management examples. By the time this adventure is over, you’ll know exactly how to use constraints to guarantee the integrity of your data..
Table of Contents
Data integrity is one of the main pillars of dependability in the broad realm of database management systems. Ensuring the accuracy, consistency, and reliability of data recorded in a database is known as data integrity. The usage of MySQL constraints is one of the most important techniques for guaranteeing data integrity in a MySQL database.
What is MySQL Constraints
Before we dive into MySQL constraints, let’s clarify the concept of data integrity. The correctness and consistency of the data in a database are referred to as data integrity. It guarantees the accuracy and dependability of the data kept in the database, which is necessary for making wise judgments and achieving significant outcomes.
MySQL Constraints are the rules enforced on the data columns of a table. These are used to limit the type of data that can go into a table. This ensures the accuracy and reliability of the data in the database.
MySQL Constraints could be either on a column level or a table level. The column level MySQL constraints are applied only to one column, whereas the table level constraints are applied to the whole table.
Databases can become untrustworthy, and their information may not be trustworthy if sufficient data integrity isn’t maintained. This is the point at which limitations apply.
MySQL Constraints are a crucial aspect of database management, as they ensure that the data entered into a database is accurate, consistent, and meets certain requirements.
Types of MySQL Constraints
MySQL constraints are rules applied to a column or a set of columns in a table. These rules define the characteristics of the data that can be stored in those columns, ensuring that it adheres to the specified conditions.
Several types of MySQL constraints can be implemented in MySQL, including primary key constraints, foreign key constraints, unique constraints, and check constraints.
Let’s see about each MySQL Constraint.
1). Primary key constraints
The Primary Key Constraint for a table enforces the table to accept unique data for a specific column, and this MySQL constraint creates a unique index for accessing the table faster.
The primary key is typically a numeric column that is automatically incremented, and it is used to link related records in different tables.
A primary key constraint ensures that the values in a specific column (or columns) are unique and not null. It’s used to uniquely identify each row in a table. Here’s an example:
CREATE TABLE students ( student_id INT PRIMARY KEY, name VARCHAR(50) );
In this example:
student_id
is declared as the primary key for thestudents
table.- It ensures that each
student_id
is unique, making it a reliable way to uniquely identify each student. - It also enforces that the
student_id
column cannot contain null values.
2). Foreign key constraints
Foreign Key in MySQL creates a link between two tables by one specific column of both tables. The specified column in one table must be a Primary Key and referred to by the column of other tables known as a Foreign Key.
For example, a foreign key constraint can be used to enforce a relationship between a customer table and an order table, ensuring that an order cannot be created without a valid customer.
A foreign key constraint establishes a relationship between tables by ensuring that data in one table’s column matches values in another table’s primary key. Let’s use an example with two tables, orders
and customers
:
CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, FOREIGN KEY (customer_id) REFERENCES customers(customer_id) );
In this example:
- The
customer_id
column in theorders
table is defined as a foreign key. - It references the
customer_id
column in thecustomers
table. - This constraint ensures that every
customer_id
in theorders
table corresponds to an existingcustomer_id
in thecustomers
table, maintaining referential integrity.
3). Unique constraints
A unique Constraint in MySQL does not allow to insertion of a duplicate value in the column. The Unique constraints maintain the uniqueness of a column in a table. More than one Unique column is used in a table.
For example, a unique constraint can be applied to an email column in a customer table, ensuring that no two customers have the same email address.
A unique key constraint, like a primary key, ensures that the values in a specific column (or columns) are unique. However, it allows for null values. Here’s an example:
CREATE TABLE employees ( employee_id INT UNIQUE, name VARCHAR(50) );
In this example:
employee_id
is declared as a unique key for theemployees
table.- It ensures that each
employee_id
is unique but allows for null values. - You can have multiple null values in this column while still enforcing uniqueness for non-null values.
4). Check constraints
A Check Constraint controls the values in the associated column. The Check Constraint determines whether the value is valid or not form a logical expression.
For example, a check constraint can be used to ensure that the value in a column is within a specific range or meets certain conditions.
In addition to the constraints mentioned above, MySQL also supports the use of default constraints, which specify a default value for a column if no value is provided.
A check constraint validates data based on a specific condition or expression. Let’s take an example with the employees
table, ensuring that salaries are positive values:
CREATE TABLE employees ( employee_id INT PRIMARY KEY, salary DECIMAL(10, 2), CHECK (salary > 0) );
In this example:
- The
salary
column has a check constraint that ensures the value in this column is greater than 0. - This constraint enforces a business rule, ensuring that salaries are always positive values.
5). Not Null Constraints:
In MySQL, Not null constraints allow to specify that a column can not contain any null value. It can be used to CREATE and ALTER a table.
The Not Null constraint ensures that a specific column does not contain null values. Here’s an example with the products
table:
CREATE TABLE products ( product_id INT PRIMARY KEY, product_name VARCHAR(50) NOT NULL );
In this example:
- The
product_name
column is defined with the not null constraint. - It ensures that every row in the
products
table must have a non-null value in theproduct_name
column, enforcing data completeness.
6). Default Constraint:
In the MySQL table, each column must contain a value. While inserting data into a table, if no value supplied to a column, then the column gets the value set as DEFAULT.
This means that if an INSERT statement does not provide a value for the column, MySQL will use the default value instead. The DEFAULT constraint is often used to ensure that a column always has a value, even if one is not explicitly provided during an INSERT operation.
Here’s an example of how to use the DEFAULT constraint in MySQL:
CREATE TABLE employees ( employee_id INT PRIMARY KEY, first_name VARCHAR(50), last_name VARCHAR(50), hire_date DATE DEFAULT '2023-01-01' );
In this example:
- We have created a table named
employees
with several columns, includingemployee_id
,first_name
,last_name
, andhire_date
. - The
hire_date
column is defined with a DEFAULT constraint. The default value is set to ‘2023-01-01’. - If an INSERT statement does not specify a value for the
hire_date
column, MySQL will automatically use ‘2023-01-01’ as the default value.
7). Auto Increment Constraints
The AUTO_INCREMENT constraint in MySQL is used to automatically generate a unique, incremental value for a column, typically used as a primary key. This is commonly used for columns that need to contain unique identifiers, such as user IDs, order numbers, or product IDs. Each time a new row is inserted into the table, MySQL automatically assigns the next sequential integer value, ensuring the uniqueness of the values.
Here’s an example of how to use the AUTO_INCREMENT constraint in MySQL:
CREATE TABLE users ( user_id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(50), email VARCHAR(100) );
In this example:
- We have created a table named
users
with three columns:user_id
,username
, andemail
. - The
user_id
column is defined with the AUTO_INCREMENT constraint and set as the primary key. - When you insert a new row into the
users
table and don’t specify a value foruser_id
, MySQL will automatically generate the next unique integer value for that column.
8). ENUM Constraint
The ENUM constraint in MySQL is used to define a column that can only contain one of a predefined set of string values. It restricts the values that can be stored in a column to a fixed list of options, making it a useful choice for columns where the data is limited to a set of specific, known values.
Here’s an example of how to use the ENUM constraint in MySQL:
CREATE TABLE colors ( color_id INT PRIMARY KEY, color_name ENUM('Red', 'Green', 'Blue', 'Yellow', 'Purple') );
In this example:
- We have created a table named
colors
with two columns:color_id
andcolor_name
. - The
color_name
column is defined with the ENUM constraint, and it can only contain one of the specified values: ‘Red’, ‘Green’, ‘Blue’, ‘Yellow’, or ‘Purple’.
9). Index Constraints
We can quickly and easily build and retrieve data from the table because to this MySQL constraint. One or more columns can be used to form an index. Each row is given a ROWID in the same manner that they were added to the table.
Here’s an example of how to create an index on a table in MySQL:
CREATE TABLE customers ( customer_id INT PRIMARY KEY, first_name VARCHAR(50), last_name VARCHAR(50), email VARCHAR(100), INDEX idx_email (email) );
In this example:
- We have created a table named
customers
with columns for customer information. - The
customer_id
column is designated as the primary key, ensuring uniqueness. - An index named
idx_email
is created on theemail
column.
Credits:
- Photo by Rubaitul Azad on Unsplash
References:
Conclusion:
In conclusion, MySQL constraints are a powerful tool for ensuring data integrity in database management. Whether you are working with a small database or a large enterprise system, constraints play a vital role in maintaining the accuracy and reliability of your data and can help to make your database management more efficient and effective.
In order to keep your database’s data integrity, MySQL constraints are essential tools. MySQL Constraints give your data the crucial structure it needs to be correct and dependable, whether they are used to enforce table relationships, guarantee data uniqueness, or validate data conditions.
By looking at the constraints on these tables, you can easily understand the rules that apply to the data. This can make it much easier to work with the database and to ensure that the data is always valid.
I hope this article has helped you to understand the power of MySQL constraints. By using constraints, you can build a better database that is more accurate, consistent, performant, and maintainable.