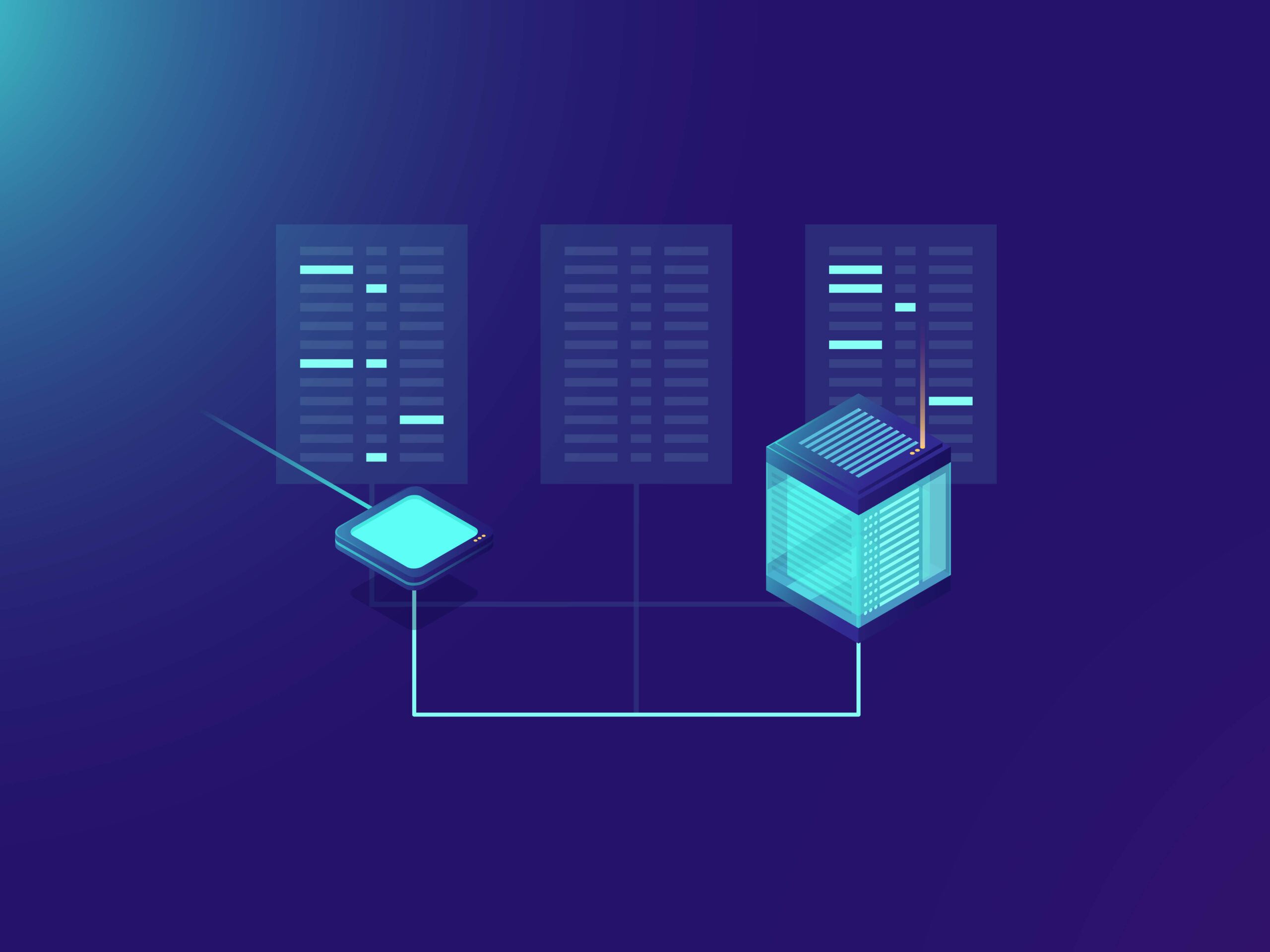
Image by fullvector on Freepik
Prisma ORM: Building Modern Data-Driven Applications in Easy Way
In this article, we’ll see about Prisma.
Table of Contents
Databases are incredibly important in the realm of software development for managing, storing, and retrieving data. However, dealing with databases directly can frequently be difficult, error-prone, and time-consuming, necessitating the creation of complicated SQL queries and the control of numerous database transactions by developers.
Object-relational mapping (ORM) technologies give a higher-level abstraction for working with databases in this situation. It is a strong competitor in this space, which offers programmers a sleek and contemporary method to interface with databases while preserving the benefits of strong typing, schema management, and performance optimization.
What is Prisma
It is an open-source ORM that makes it fun and safe to work with a database like MySQL, Postgres, SQL Server, or MongoDB.
It is an open-source ORM for Node.js and TypeScript. It is used as an alternative to writing plain SQL or using another database access tool such as SQL query builders (like knex.js) or ORMs (like TypeORM and Sequelize). Currently, it supports PostgreSQL, MySQL, SQL Server, SQLite, MongoDB, and CockroachDB (Preview).
It simplifies database operations by allowing developers to work with databases using a more intuitive and type-safe approach, eliminating much of the traditional SQL boilerplate code.
It consists of the following parts:
- Prisma Client: Auto-generated and type-safe query builder for Node.js & TypeScript
- Prisma Migrate: Migration system
- Prisma Studio: GUI to view and edit data in your database.
It unlocks a new level of developer experience when working with databases thanks to its intuitive data model, automated migrations, type-safety & auto-completion.
Features:
- Database Schema Modeling: It allows you to define your database schema using a Domain-Specific Language (DSL). This schema definition is written in a Prisma-specific syntax that defines your data models, fields, relationships, and constraints.
- Type-Safe Queries: It generates type-safe query builders based on your schema definition. This means that you can write queries using your preferred programming language (e.g., TypeScript or Rust) and get compile-time validation, catching errors early in the development process.
- CRUD Operations: It supports basic CRUD (Create, Read, Update, Delete) operations on your database models. You can create, retrieve, update, and delete records from your database using the generated query methods.
- Relationships: It supports defining and working with relationships between different data models, such as one-to-one, one-to-many, and many-to-many relationships. The ORM handles the complexity of managing these relationships.
- Migrations: It helps manage database schema changes over time through a migrations system. You can version-control and apply schema changes using commands, ensuring that your database schema evolves alongside your application.
- Data Validation: It performs data validation based on the schema definition, ensuring that the data you insert or update conforms to the expected structure and constraints.
- Performance: It optimizes database queries and minimizes the “N+1 query” problem by batching related queries and fetching only the necessary data.
- Database Agnostic: It supports multiple database systems, including PostgreSQL, MySQL, SQLite, and SQL Server, allowing you to switch between these databases with minimal code changes.
- Authentication and Authorization: It can be integrated with authentication and authorization systems, allowing you to secure your database access and operations.
- Real-time Capabilities: It can be combined with other tools (like WebSocket libraries) to enable real-time features, such as live updates and notifications.
Installation with Example:
Before starting the installation, make sure you have already installed node.js and MySQL on your device. In this example, we’ll create a simple Node.js application based on Javascript that uses Prisma to interact with a MySQL database.
Step 1: Start by installing the Prisma CLI globally using npm:
npm install -g prisma
Step 2: In your terminal, navigate to the root directory of your project and run the following command to initialize a new Prisma project:
npx prisma init
Step 3: Open the .env file and configure the MySQL database URL. for example, see below:
DATABASE_URL="mysql://root:@localhost:3306/prismadb"
Step 4: Open the schema.prisma file in your project directory and define your data models. You need to also connect your database configuration here. For this example, let’s define a simple User model:
// schema.prisma datasource db { provider = "mysql" url = "mysql://root:@localhost:3306/prismadb" } model User { id Int @id @default(autoincrement()) username String @unique email String @unique }
Step 5: Run the following command to generate the Prisma Client based on your schema:
npx prisma generate
Step 6: If you make changes to your schema, you’ll need to generate a migration and apply it to the database:
npx prisma migrate dev --name init
This command does two things:
It creates a new SQL migration file for this migration
It runs the SQL migration file against the database
This ensures that your database schema stays in sync with your Prisma schema.
Step 7: In your project directory, initialize a new npm project (if you haven't already) and install the required dependencies: npm init -y npm install @prisma/client
Step 8: Now, let’s create a simple JavaScript file to demonstrate how to create and query data using Prisma.
Create a file called “app.js”
// app.js const { PrismaClient } = require('@prisma/client'); const prisma = new PrismaClient(); async function main() { // Create a new user const newUser = await prisma.user.create({ data: { username: 'john_doe', email: 'john@example.com', }, }); console.log('New user created:', newUser); // Query all users const allUsers = await prisma.user.findMany(); console.log('All users:', allUsers); } main() .catch((error) => { console.error('Error:', error); }) .finally(async () => { await prisma.$disconnect(); });
Step 9: Run the application using Node.js:
node app.js
You’ll see the output of the newly created user and the list of all users queried from the database.
Great! In this example, you learned how to install and integrate It with a JavaScript application. It simplifies database interactions, provides type-safe queries, and offers a clean and intuitive API for managing databases.
You can follow the below video for the installation process with a sample example:
Use cases:
Prisma ORM can be used for a variety of applications and situations, including:
- Web Application: It is a good choice for developing online apps that need reliable data storage and retrieval. Applications having a lot of database interactions can benefit especially from its performance optimization features.
- API Development: When developing APIs that communicate with databases, It’s type-safe queries can be used to make sure that the replies from the API match the desired data structure.
- Microservices: Each service frequently has its own database in a microservices design. It is simpler to manage and evolve databases across diverse services thanks to Its’s database agnosticism and migrations support.
- Real-Time Applications: Applications that deliver real-time updates and notifications to users can be created by developers by integrating it with real-time communication tools.
- Prototyping and MVPs: Prisma makes early-stage database interactions simpler so that developers can concentrate on creating key features rather than dealing with challenging SQL queries. This enables prototyping and MVPs.
Advantages and Disadvantages:
Advantages:
- Type Safety: It provides type-safe query builders, which means you catch errors at compile time rather than runtime.
- Schema Management: The schema definition acts as a single source of truth for your data models, making schema changes and evolutions easier to manage.
- Performance Optimization: It optimizes queries by batching and fetching only the necessary data, improving performance.
- Code Maintainability: It reduces the amount of boilerplate code, leading to cleaner and more maintainable codebases.
- Cross-Database Compatibility: It supports multiple database systems, allowing you to switch databases with minimal changes to your application code.
Disadvantages:
- Learning Curve: When adjusting to the DSL and comprehending how to deal with the generated query builders, developers who are new to It may encounter a learning curve.
- Schema Evolution: Managing schema changes and migrations becomes crucial as the application develops. Smooth transitions must be planned for and tested carefully.
- Complex Queries: While It streamlines the majority of database interactions, sophisticated queries that fall outside of the norm may need further modification.
- Performance tuning: Even though It offers speed improvements, programmers should be mindful of the possibility of performance bottlenecks in their programs.
Reference:
- https://docs.nestjs.com/recipes/prisma
- https://www.prisma.io/docs/getting-started/setup-prisma/start-from-scratch/relational-databases-node-mysql
Credits:
- Image by fullvector on Freepik
Conclusion:
With regard to database interactions in contemporary software development, It offers a novel perspective. It enables developers to easily create strong and maintainable systems by fusing the power of type-safe queries, schema creation, and performance optimization.
As the software industry develops, technologies like Prisma are essential for streamlining difficult operations and freeing developers from the complexities of database management so they can concentrate on developing novel and effective solutions.
It’s features and advantages make it an appealing option for streamlining your database interactions and elevating your projects, whether you’re developing web applications, APIs, or microservices.