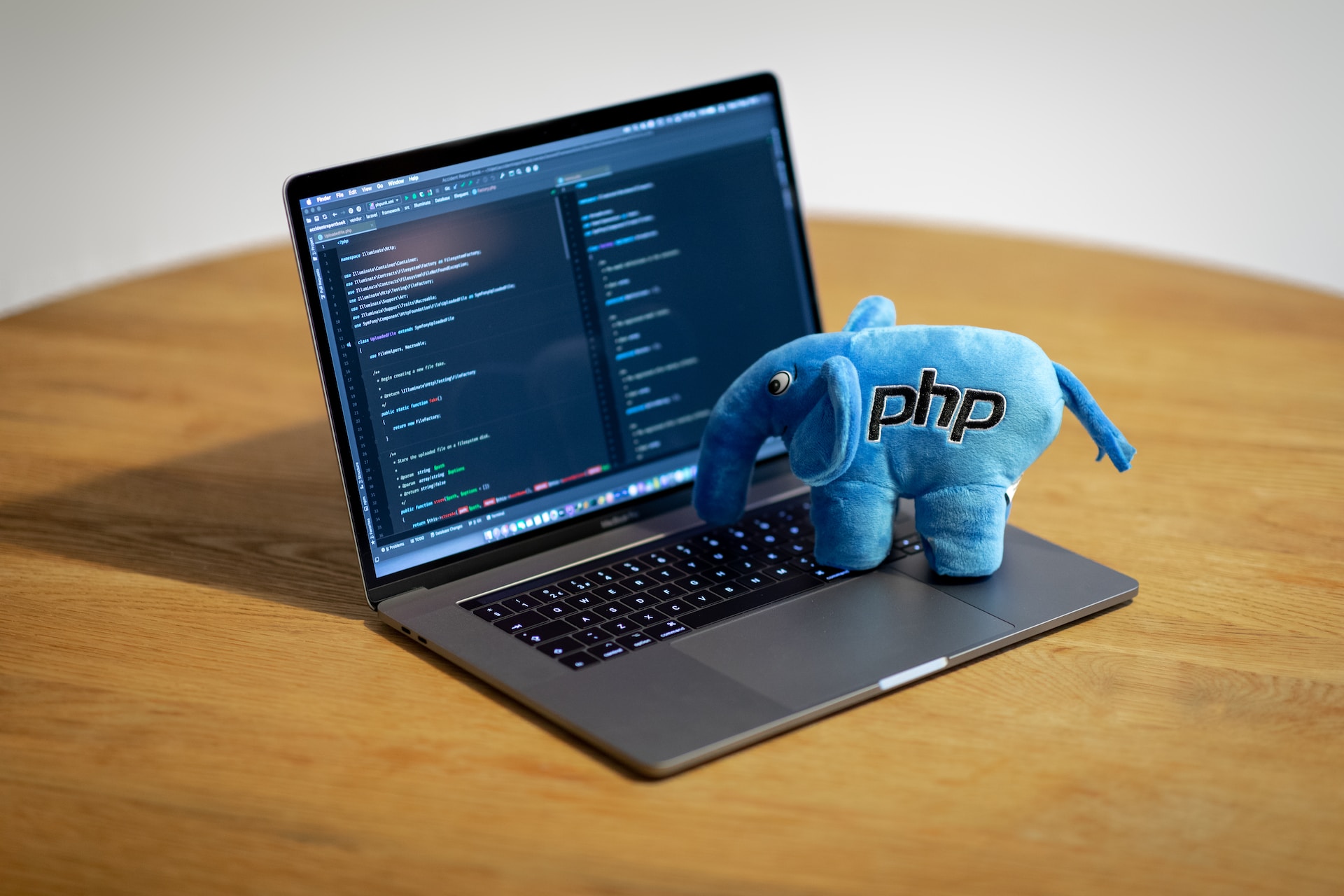
Easy Way to Find the Smallest Number in Array in PHP
In this article, we will write a Program to Find the smallest number in Array.
Table of Contents
Arrays play a vital role in programming, allowing us to organize and manipulate collections of elements. At times, we encounter situations where we need to uncover the smallest number in array.
While it may sound like a simple task, it’s essential to approach it with an optimized solution. In this blog post, we’ll introduce you to a user-friendly program that effortlessly finds the smallest number in array.
Let’s dive in and unravel the tiniest gem!
Arrays are complex variables that allow us to store more than one value or a group of values under a single variable name. An array is an special variable which can hold more than one value at a time.
An array is a special variable, which can hold more than one value at a time.
If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
$cars1 = “Volvo”;
$cars2 = “BMW”;
$cars3 = “Toyota”;
However, what if you want to loop through the cars and find a specific one? And what if you had not 3 cars, but 300?
The solution is to create an array!
An array can hold many values under a single name, and you can access the values by referring to an index number.
Program to Find the smallest number in Array
We will discuss two different methods: the brute-force method and the min() function.
The Brute-Force Method
The brute-force method is the simplest method to find the smallest number in array. It works by simply comparing each element of the array to the current smallest number. The element that is smaller than the current smallest number becomes the new smallest number.
The following code shows an example of the brute-force method:
<?php // Initialize the array $arr = [10, 5, 2, 7, 3]; // Initialize the smallest number $smallest = $arr[0]; // Loop through the array foreach ($arr as $number) { // Compare the current element to the smallest number if ($number < $smallest) { // Update the smallest number $smallest = $number; } } // Print the smallest number echo "The smallest number is $smallest"; ?>
The min() Function
The min() function is a more efficient method to find the smallest number in array. It works by looping through the array and returning the smallest element.
The following code shows an example of the min() function:
<?php // Initialize the array $arr = [10, 5, 2, 7, 3]; // Get the smallest number using the min() function $smallest = min($arr); // Print the smallest number echo "The smallest number is $smallest"; ?>
Example Program
Following is a PHP program to find the minimum number from an array:
<?php $numbers=array(12,23,45,20,5,6,34,17,9,56); $length=count($numbers); $min=$numbers[0]; for($i=1;$i<$length;$i++) { if($numbers[$i]<$min) { $min=$numbers[$i]; } } echo "The smallest number is ".$min; ?>
PHP also has an inbuilt min() function. The min() function returns the lowest value in an array or the lowest value of several specified values. See the below example:
<?php echo min(2, 3, 1, 6, 7); // 1 echo min(array(2, 4, 5)); // 2 ?>
Conclusion:
With our user-friendly program, finding the smallest number in array becomes a breeze. By effortlessly traversing the elements and meticulously comparing each one, we can uncover the true gem hiding in the array.
This simple yet powerful tool can be easily adapted to suit your preferred programming language, making it a valuable asset in your coding endeavors.
Remember, arrays are like treasure troves of data, and mastering their manipulation is a fundamental skill in programming.
By sharpening your ability to find the smallest number in array, you not only enhance your problem-solving skills but also lay a solid foundation for tackling more intricate programming challenges in the future.
Embrace the journey of coding, and may you discover countless gems along the way! Happy coding!
I hope this article helps!