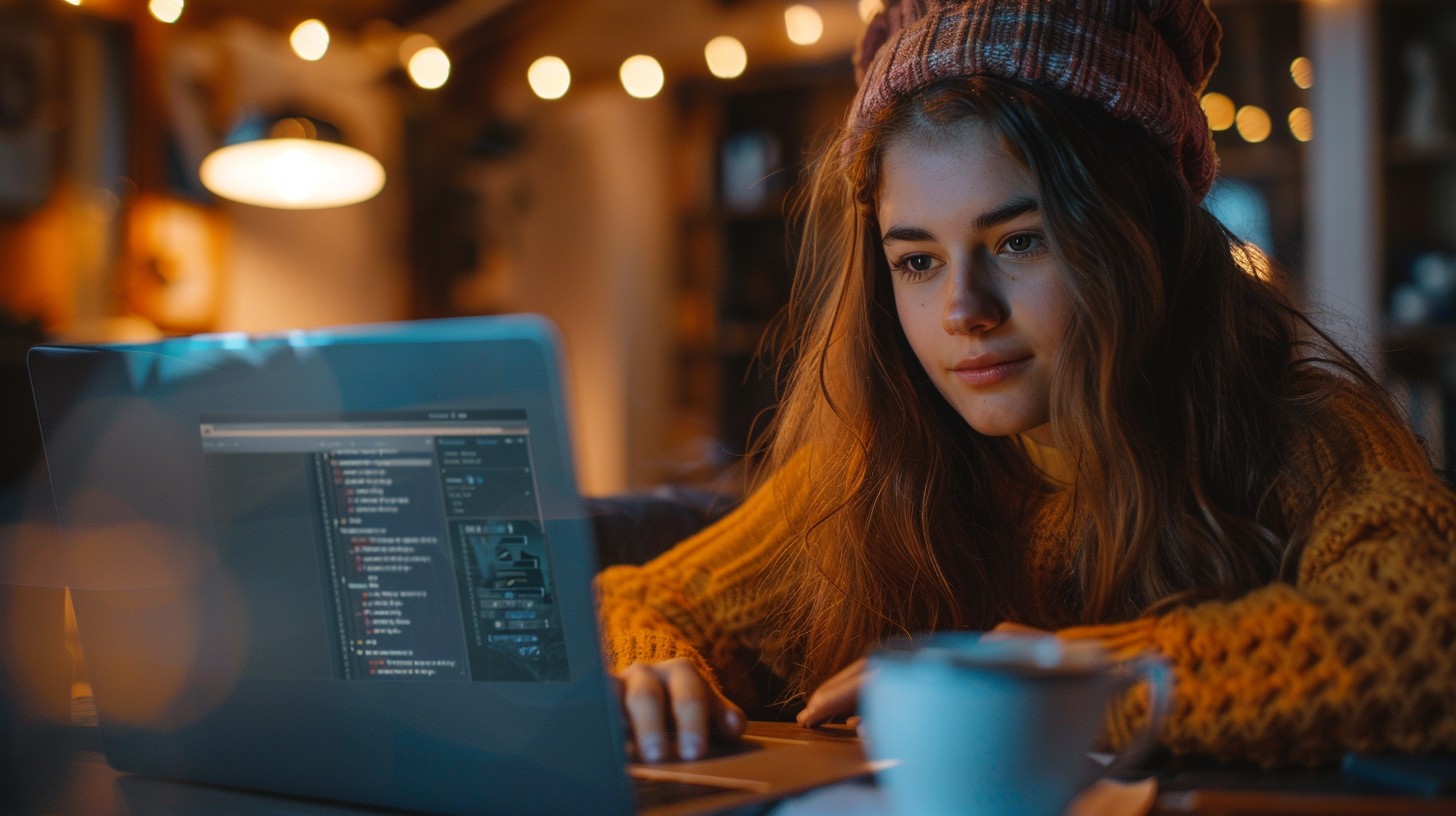
Python Variables : Understanding Scope, Lifetime, and Best Practices
Python Variables play a crucial role in coding by enabling the storage and modification of information. In Python, variables are remarkably adaptable and straightforward, rendering Python an excellent choice for both novices and seasoned programmers.
Table of Contents
This article will cover the basics of Python variables, how to use them, and some best practices to keep in mind.
What are Python Variables
Python variables are used to store values. Whenever we want to store any value in Python, we use Variables. In simple words Variable acts like a container to store any value in it, It can be any number, any text, or a combination of both.
This value can be of various data types, such as numbers, strings, lists, or even more complex data structures. Think of a variable as a labeled box where you can store data to be used later in your code.
Creating Variables in Python
Creating a variable in Python is straightforward. You use the assignment operator (=
) to assign a value to a variable name.
x = 5 name = "Alice" is_student = True
In this example:
x
is an integer variable with a value of 5.name
is a string variable with a value of “Alice”.is_student
is a boolean variable with a value ofTrue
.
Python Variables Naming Rules
A Python variables name must follow these rules:
- Names can contain letters, digits, and underscores:
my_variable
,var123
,name_1_2_3
. - Names must start with a letter or an underscore:
_variable
,variable1
, but not1variable
. - Names are case-sensitive:
myVariable
andmyvariable
are two different variables. - Avoid using Python-reserved words: Words like
class
,for
, andif
have special meanings in Python and should not be used as variable names.
Examples of Valid and Invalid Variable Names
# Valid variable names my_variable = 10 _var = 20 name1 = "John" # Invalid variable names 1variable = 10 # Starts with a digit class = "Hello" # Reserved word my-variable = 30 # Contains a hyphen
Dynamic Typing in Python
Python is a dynamically typed language, which means you don’t have to declare the type of a variable when you create it. The type is inferred from the value assigned to it. This allows for great flexibility but requires careful management to avoid unexpected behavior.
x = 5 x = "Now I'm a string"
In the above example, x
starts as an integer but is later reassigned to a string. This flexibility is powerful but can lead to unexpected behavior if not managed carefully.
Checking Variable Types
You can check the type of a variable using the built-in type()
function:
x = 5 print(type(x)) # <class 'int'> x = "Hello" print(type(x)) # <class 'str'>
Common Data Types
Python supports several built-in data types, including:
- Integers: Whole numbers, e.g.,
5
,-3
. - Floats: Decimal numbers, e.g.,
3.14
,-2.0
. - Strings: Text, e.g.,
"Hello, world!"
. - Booleans: Truth values,
True
andFalse
. - Lists: Ordered collections, e.g.,
[1, 2, 3]
. - Dictionaries: Key-value pairs, e.g.,
{'name': 'Alice', 'age': 25}
. - Tuples: Ordered, immutable collections, e.g.,
(1, 2, 3)
. - Sets: Unordered collections of unique elements, e.g.,
{1, 2, 3}
.
Example Usage of Different Data Types
# Integer age = 30 # Float height = 5.9 # String name = "Alice" # Boolean is_student = True # List colors = ["red", "blue", "green"] # Dictionary person = {"name": "Alice", "age": 25} # Tuple coordinates = (10.0, 20.0) # Set unique_numbers = {1, 2, 3}
Object References in Python
In Python, variables are references to objects stored in memory. This means that variables do not hold the actual data themselves; instead, they point to the location in memory where the data is stored.
Example of Object References
a = [1, 2, 3] b = a print(a) # Output: [1, 2, 3] print(b) # Output: [1, 2, 3] a.append(4) print(a) # Output: [1, 2, 3, 4] print(b) # Output: [1, 2, 3, 4]
Explanation:
- a = [1, 2, 3]:
a
references a list object. - b = a:
b
references the same list object asa
. - a.append(4): Modifies the list object in place. Since
a
andb
reference the same object, both see the change.
This demonstrates that variables are references to objects, and changes to the object through one reference are reflected in all references to that object.
Object Identity in Python
Every object in Python has a unique identity that can be accessed using the id()
function. The identity is a unique integer that remains constant for the object during its lifetime. The is
operator can be used to test whether two variables reference the same object.
Example of Object Identity
x = [1, 2, 3] y = [1, 2, 3] z = x print(id(x)) # Outputs the unique identity of the list referenced by x print(id(y)) # Outputs the unique identity of the list referenced by y print(id(z)) # Outputs the same identity as x, since z references the same object print(x is y) # Output: False, because x and y reference different objects print(x is z) # Output: True, because x and z reference the same object
Explanation:
- id(x): Returns the unique identity of the list object referenced by
x
. - id(y): Returns the unique identity of a different list object, even though it has the same contents as
x
. - id(z): Returns the same identity as
x
, becausez
references the same object asx
. - x is y: Returns
False
becausex
andy
reference different objects. - x is z: Returns
True
becausex
andz
reference the same object.
Understanding object identity helps in determining whether two variables reference the same object or different objects with the same value. This is especially important when working with mutable objects.
Type Conversion
Python provides built-in functions to convert variables from one type to another. Common functions include int()
, float()
, str()
, and bool()
.
x = "123" y = int(x) # y is now an integer with value 123 z = float(x) # z is now a float with value 123.0
Explanation:
- x = “123”: A string variable representing a number.
- y = int(x): Converts the string
x
to an integer, resulting iny = 123
. - z = float(x): Converts the string
x
to a float, resulting inz = 123.0
.
Variable Scope
The scope of Python variables refers to the region of the code where the variable is recognized. In Python, variables can have:
- Local Scope: Variables defined inside a function. They can only be used within that function.
- Global Scope: Variables defined outside any function. They can be accessed from anywhere in the code.
Example of Variable Scope
global_var = "I'm global" def my_function(): local_var = "I'm local" print(global_var) # This will work print(local_var) # This will work print(global_var) # This will work print(local_var) # This will cause an error
Explanation:
- global_var = “I’m global”: A global variable that can be accessed anywhere in the code.
- local_var = “I’m local”: A local variable that is only accessible within the
my_function
function. - print(global_var): This works both inside and outside the function because
global_var
is a global variable. - print(local_var): This works inside the function but causes an error outside because
local_var
is local tomy_function
.
Global Keyword
If you need to modify a global variable inside a function, you can use the global
keyword.
x = 5 def my_function(): global x x = 10 my_function() print(x) # Output: 10
Avoid Using Python Reserved Word
Python has a set of reserved words, also known as keywords, that have special meanings in the language. These words are part of the syntax and cannot be used as variable names. Here are some common reserved words in Python:
and as assert break class continue def del elif else except False finally for from global if import in is lambda None nonlocal not or pass raise return True try while with yield
Explanation:
- Reserved words are predefined and have special significance in the Python language.
- Using these words as variable names will result in a syntax error because Python will not be able to distinguish between the intended use of the word and its special meaning.
Credits:
- Photo by Stockcake
Conclusion
Python Variables are a cornerstone of programming in Python. Understanding how to effectively use Python variables, including their creation, naming, and scope, is crucial for writing clear and efficient code. By adhering to best practices and leveraging Python’s dynamic typing, you can make the most of this powerful language feature.
Mastering Python variables lays a solid foundation for tackling more complex programming concepts and challenges. Happy coding!