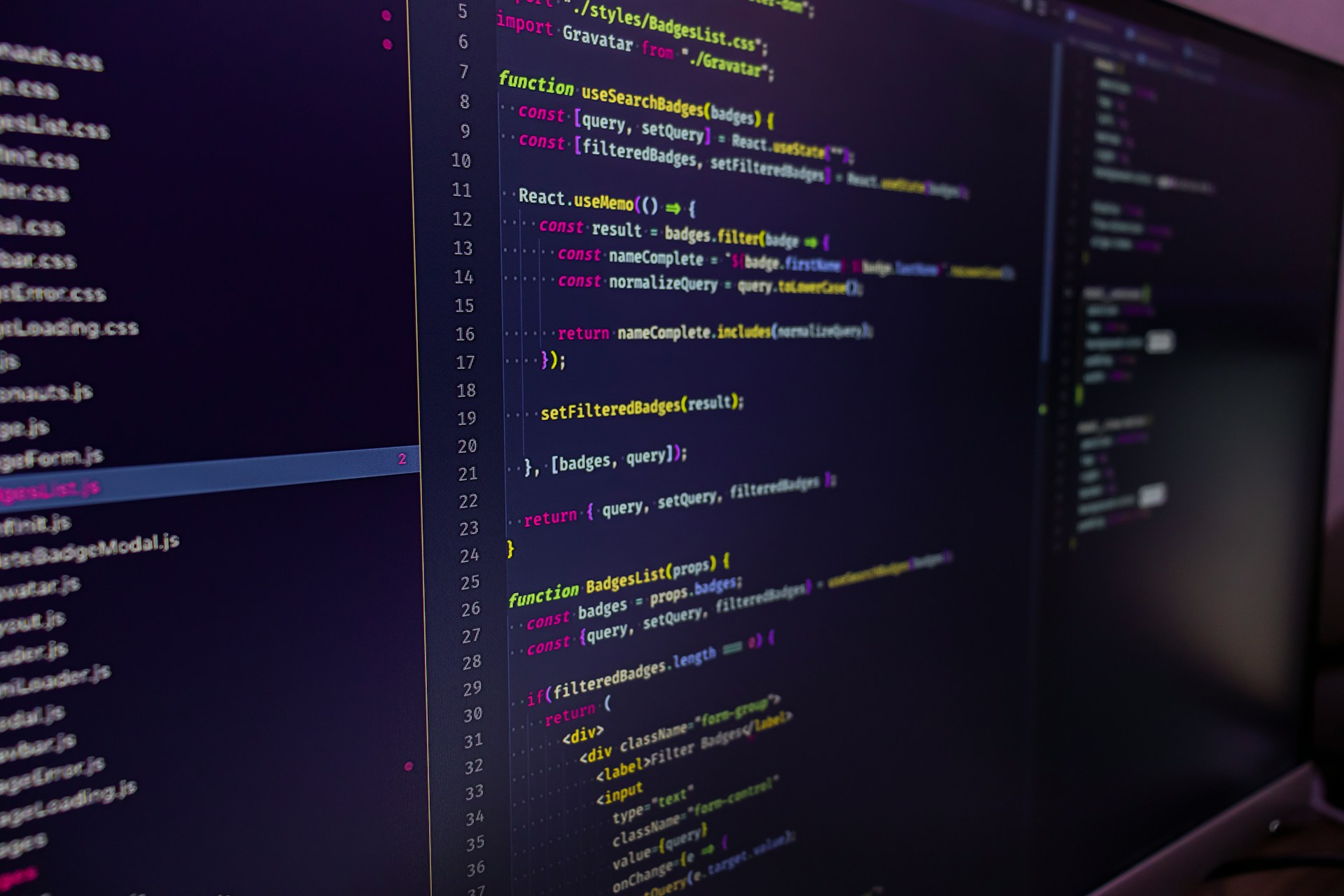
React Forms : Building Powerful User Interactions
React Forms are a crucial component of react-based web applications, facilitating user interaction and gathering information. React, a popular JavaScript library for building user interfaces provides a robust approach to creating dynamic and interactive forms.
Table of Contents
In this article, we’ll learn about React forms basics, top tips, and sophisticated methods for handling React forms. By the conclusion of this piece, you’ll possess a thorough grasp of how to develop strong, sustainable, and appealing forms.
What are React Forms
Forms are essential for capturing user input, allowing users to interact with your application by submitting data.
React forms are essential components that leverage HTML form elements to capture user input. However, unlike traditional HTML forms that manage their state directly in the DOM, React forms employ a stateful approach. This means that the form’s state, representing the values entered by the user, is maintained within the React component itself.
React forms can be managed using controlled or uncontrolled components. Understanding these two approaches is crucial for building effective React forms.
Controlled vs. Uncontrolled Components
Controlled Components:
In controlled components, form data is handled by the component’s state. This means the input values are always in sync with the component state, providing better control and validation.
The recommended approach for building React forms is through the use of controlled components. In controlled components, the form’s state (the values of input fields) is directly managed by the React component. This is achieved by:
- Initializing State: The component’s state object is initialized with properties corresponding to each form field. These properties will hold the current values entered by the user.
- Binding Values to Inputs: The
value
attribute of each input element within the form is bound to the corresponding state property using JavaScript expressions (JSX syntax). - Handling User Input: Event handlers, typically attached to the
onChange
event of input elements, are used to capture user input. These handlers update the component’s state using thesetState
method, reflecting the changes in the UI.
Uncontrolled Components:
While controlled components are generally preferred, uncontrolled components can be useful in specific scenarios where managing state directly in the DOM might be simpler. However, uncontrolled components can make handling form data and validation more challenging.
This section demonstrates the difference between controlled and uncontrolled components with clear, practical examples to help you understand when to use each approach in your React forms.
import React, { useState, useRef } from 'react'; const ControlledForm = () => { const [value, setValue] = useState(''); const handleChange = (event) => { setValue(event.target.value); }; const handleSubmit = (event) => { event.preventDefault(); alert(`Submitted value: ${value}`); }; return ( <form onSubmit={handleSubmit}> <input type="text" value={value} onChange={handleChange} /> <button type="submit">Submit</button> </form> ); }; const UncontrolledForm = () => { const inputRef = useRef(null); const handleSubmit = (event) => { event.preventDefault(); alert(`Submitted value: ${inputRef.current.value}`); }; return ( <form onSubmit={handleSubmit}> <input type="text" ref={inputRef} /> <button type="submit">Submit</button> </form> ); }; export { ControlledForm, UncontrolledForm };
In this example, ControlledForm uses state to manage input value, while UncontrolledForm uses a ref to access the input value.
Basic Form Handling
In this section, we delve into creating a more detailed controlled form example with multiple fields and a submit button. You’ll learn how to manage form state effectively and handle user input seamlessly in React forms.
import React, { useState } from 'react'; const BasicForm = () => { const [formData, setFormData] = useState({ name: '', email: '', password: '', }); const handleChange = (event) => { const { name, value } = event.target; setFormData({ ...formData, [name]: value, }); }; const handleSubmit = (event) => { event.preventDefault(); console.log('Form Data Submitted:', formData); }; return ( <form onSubmit={handleSubmit}> <label> Name: <input type="text" name="name" value={formData.name} onChange={handleChange} /> </label> <br /> <label> Email: <input type="email" name="email" value={formData.email} onChange={handleChange} /> </label> <br /> <label> Password: <input type="password" name="password" value={formData.password} onChange={handleChange} /> </label> <br /> <button type="submit">Submit</button> </form> ); }; export default BasicForm;
In this BasicForm component, we use a state object to manage multiple input fields. The handleChange function updates the state based on the input name and value.
React Forms Validation Techniques
Form validation is crucial for ensuring data integrity and providing feedback to users. In this section, we cover various validation techniques, including inline validation, custom validation functions, and using libraries like Yup for schema-based validation. Learn how to implement robust validation in your React forms to enhance user experience.
Inline Validation:
Inline validation checks the input values directly within the form submission handler. This method is straightforward and effective for simple forms but can become unwieldy for complex forms.
const handleSubmit = (event) => { event.preventDefault(); if (!formData.name || !formData.email || !formData.password) { alert('All fields are required'); } else { console.log('Form Data Submitted:', formData); } };
In this example, the handleSubmit function checks if any of the fields are empty and alerts the user if they are. Otherwise, it logs the submitted form data.
Custom Validation Functions:
Custom validation functions provide more flexibility and can handle complex validation logic. These functions can validate multiple fields and provide detailed error messages.
const validateForm = () => { const errors = {}; if (!formData.name) { errors.name = 'Name is required'; } if (!formData.email) { errors.email = 'Email is required'; } else if (!/\S+@\S+\.\S+/.test(formData.email)) { errors.email = 'Email address is invalid'; } if (!formData.password) { errors.password = 'Password is required'; } else if (formData.password.length < 6) { errors.password = 'Password must be at least 6 characters'; } return errors; }; const handleSubmit = (event) => { event.preventDefault(); const errors = validateForm(); if (Object.keys(errors).length === 0) { console.log('Form Data Submitted:', formData); } else { console.log('Validation Errors:', errors); } };
Here, the validateForm function checks each field and returns an object containing any validation errors. The handleSubmit function then checks if there are any errors before submitting the form data.
Using Libraries for Validation:
Libraries like Yup
can simplify validation with schema-based validation:
import * as Yup from 'yup'; const validationSchema = Yup.object().shape({ name: Yup.string().required('Name is required'), email: Yup.string().email('Invalid email').required('Email is required'), password: Yup.string().min(6, 'Password must be at least 6 characters').required('Password is required'), }); const handleSubmit = (event) => { event.preventDefault(); validationSchema .validate(formData, { abortEarly: false }) .then(() => { console.log('Form Data Submitted:', formData); }) .catch((err) => { console.log('Validation Errors:', err.errors); }); };
In this example, we use Yup to define a validation schema. The handleSubmit function uses this schema to validate the form data. If validation passes, the form data is logged; otherwise, validation errors are logged.
Using Custom Hooks for Form Handling
Custom hooks can encapsulate form logic, making components cleaner and more reusable. This section demonstrates how to create and use custom hooks to handle form state and validation in React forms, promoting code reuse and maintainability.
Creating the Custom Hook:
import { useState } from 'react'; const useForm = (initialValues) => { const [values, setValues] = useState(initialValues); const handleChange = (event) => { const { name, value } = event.target; setValues({ ...values, [name]: value, }); }; const resetForm = () => { setValues(initialValues); }; return [values, handleChange, resetForm]; }; export default useForm;
The useForm custom hook abstracts the form state management logic. It takes initialValues as an argument and returns the current form values, a handleChange function to update the state, and a resetForm function to reset the form to its initial state. This hook can be used across multiple form components, ensuring consistency and reducing boilerplate code.
Using this custom hook in a form component:
import React from 'react'; import useForm from './useForm'; const HookForm = () => { const [formData, handleChange, resetForm] = useForm({ name: '', email: '', password: '', }); const handleSubmit = (event) => { event.preventDefault(); console.log('Form Data Submitted:', formData); resetForm(); }; return ( <form onSubmit={handleSubmit}> <label> Name: <input type="text" name="name" value={formData.name} onChange={handleChange} /> </label> <br /> <label> Email: <input type="email" name="email" value={formData.email} onChange={handleChange} /> </label> <br /> <label> Password: <input type="password" name="password" value={formData.password} onChange={handleChange} /> </label> <br /> <button type="submit">Submit</button> </form> ); }; export default HookForm;
In the HookForm component, we utilize the useForm custom hook to manage the form state. The handleChange function updates the form state whenever an input field changes. The handleSubmit function handles form submission, logs the form data, and resets the form to its initial state. This approach makes the component code cleaner and more manageable.
Advanced Form Management with Third-Party Libraries
For more complex forms, consider using libraries like Formik and React Hook Form. These libraries offer advanced features such as validation schemas, field arrays, and better performance.
This section provides examples and explanations on how to integrate and use these libraries in your React forms.
Using Formik:
import React from 'react'; import { Formik, Form, Field, ErrorMessage } from 'formik'; import * as Yup from 'yup'; const validationSchema = Yup.object().shape({ name: Yup.string().required('Name is required'), email: Yup.string().email('Invalid email').required('Email is required'), password: Yup.string().min(6, 'Password must be at least 6 characters').required('Password is required'), }); const FormikForm = () => { const initialValues = { name: '', email: '', password: '', }; const handleSubmit = (values) => { console.log('Form Data Submitted:', values); }; return ( <Formik initialValues={initialValues} validationSchema={validationSchema} onSubmit={handleSubmit}> {({ isSubmitting }) => ( <Form> <label> Name: <Field type="text" name="name" /> <ErrorMessage name="name" component="div" /> </label> <br /> <label> Email: <Field type="email" name="email" /> <ErrorMessage name="email" component="div" /> </label> <br /> <label> Password: <Field type="password" name="password" /> <ErrorMessage name="password" component="div" /> </label> <br /> <button type="submit" disabled={isSubmitting}>Submit</button> </Form> )} </Formik> ); }; export default FormikForm;
In the FormikForm component, we use the Formik component to handle the form state and validation. The Formik component wraps around the form elements and provides props such as initialValues, validationSchema, and onSubmit to manage form data, validation, and submission. The Field component is used for each input field, and ErrorMessage is used to display validation errors.
Using React Hook Form:
import React from 'react'; import { useForm } from 'react-hook-form'; const ReactHookForm = () => { const { register, handleSubmit, errors } = useForm(); const onSubmit = (data) => { console.log('Form Data Submitted:', data); }; return ( <form onSubmit={handleSubmit(onSubmit)}> <label> Name: <input name="name" ref={register({ required: 'Name is required' })} /> {errors.name && <p>{errors.name.message}</p>} </label> <br /> <label> Email: <input name="email" ref={register({ required: 'Email is required', pattern: { value: /\S+@\S+\.\S+/, message: 'Invalid email' } })} /> {errors.email && <p>{errors.email.message}</p>} </label> <br /> <label> Password: <input name="password" type="password" ref={register({ required: 'Password is required', minLength: { value: 6, message: 'Password must be at least 6 characters' } })} /> {errors.password && <p>{errors.password.message}</p>} </label> <br /> <button type="submit">Submit</button> </form> ); }; export default ReactHookForm;
In the ReactHookForm component, we use the useForm hook from the react-hook-form library to manage form state and validation. The register function is used to register each input field with validation rules. The handleSubmit function handles form submission, and the errors object provides validation error messages.
Credits:
- Photo by Fili Santillán on Unsplash
Conclusion:
In conclusion, becoming proficient in React forms requires grasping controlled and uncontrolled components, making use of custom hooks, incorporating third-party libraries, and adhering to recommended guidelines to prevent typical issues.
By applying these methods, you can develop sturdy, easy-to-manage React forms that elevate the user experience of your web projects.
Keep in mind that React forms play a crucial role in every web application, and managing them efficiently can greatly enhance the general quality and usability of your app. Enjoy your coding journey!