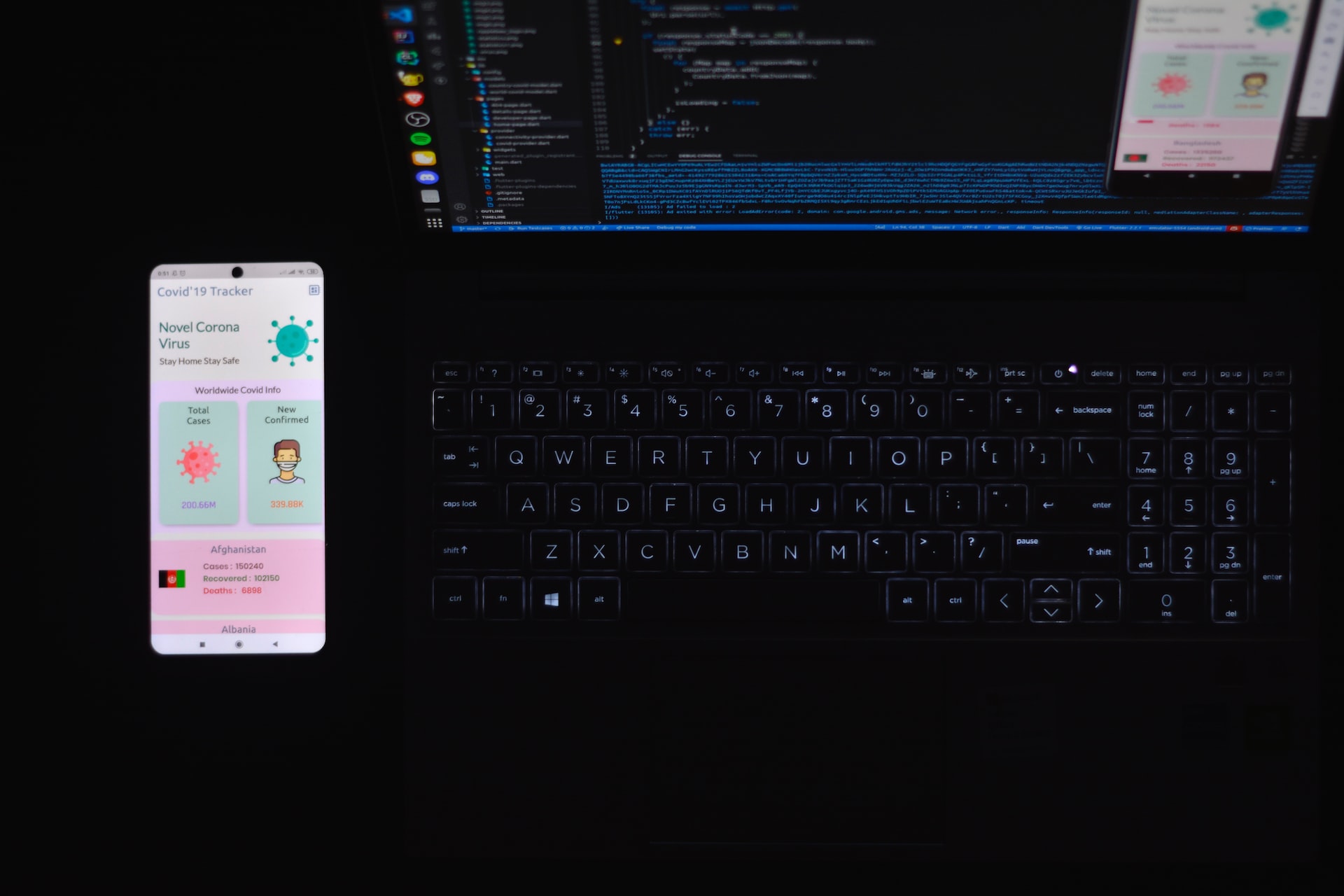
WordPress REST API
In this article, we’ll know about WordPress REST API.
Table of Contents
What is WordPress Rest API?
The WordPress REST API is a powerful tool that allows developers to access and manipulate data from WordPress websites using simple HTTP requests. The REST API is built on top of the WordPress core and provides a simple, standardized interface for accessing and manipulating data.
Rest API stands for REpresentational State Transfer Application Programming Interface. It provides a way to retrieve pure data( usually in JSON or XML format) over HTTP.
The REST API uses JSON (JavaScript Object Notation) as its data format, making it easy to parse and consume with virtually any programming language or platform. It also supports OAuth authentication, which allows users to securely grant third-party applications access to their WordPress site without sharing their login credentials. The WordPress REST API allows applications to interact with your WordPress site by sending and receiving data as JSON (JavaScript Object Notation) objects.
The API is a key tool that developers can use to easily share data from their WordPress website to other websites or applications. To use the REST API, you simply need to know how to interact with it, which boils down to using four different types of HTTP methods as part of your requests:
- GET: With this method, you can fetch information from the server.
- POST: This enables you to send information to the server in question.
- PUT: With the put method, you can edit and update existing data.
- DELETE: This enables you to delete information.
Features
The WordPress REST API offers several features that make it a powerful tool for developers:
- Standardization: It follows the industry-standard principles of Representational State Transfer (REST), making it easier to understand and use.
- Flexibility: It provides access to all WordPress data, allowing developers to build custom applications and integrations.
- Security: It uses WordPress authentication mechanisms, ensuring that only authorized users can access the data.
- Performance: It is designed to be fast and efficient, minimizing the load on the server and reducing response times.
- Extensibility: It is highly extensible, allowing developers to create custom endpoints and modify the default behavior.
How to Use the WordPress REST API
To use it, You can make HTTP requests to the API endpoints using any programming language or tool that supports HTTP requests. Here are the steps you need to follow:
- Enable the REST API: By default, It is enabled in WordPress. However, if you are using an older version of wordpress (Older than 4.7), you may need to install and activate the REST API plugin.
- Authenticate the user: To access the REST API, you need to authenticate the user. You can use the built-in authentication mechanism of wordpress or create a custom authentication method.
- Make HTTP requests: Once you have authenticated the user, you can make HTTP requests to the REST API endpoints. You can use the GET, POST, PUT, DELETE, and other HTTP methods to perform various operations on wordpress data.
- Handle the response: After making the HTTP request, you will receive a response from the API. You can handle the response in your code and parse the data to display it on your website.
Examples of Using the WordPress REST API
See below sample example of how you can use the WordPress REST API to display posts on an external website using JavaScript
// Define the API endpoint
const apiEndpoint = 'https://your-wordpress-site.com/wp-json/wp/v2/posts';
// Fetch the posts using the API endpoint
fetch(apiEndpoint) .then(response => response.json()) .then(posts => {
// Loop through the posts and display them on the webpage
posts.forEach(post => {
const postElement = document.createElement('div');
postElement.innerHTML = ` <h2>${post.title.rendered}</h2> <p>${post.excerpt.rendered}</p> <a href="${post.link}">Read More</a> `;
document.getElementById('posts-container').appendChild(postElement); });
}) .catch(error => console.error(error));
In this example, we first define the API endpoint for the WordPress site’s posts. We then use the fetch
function to make a GET request to the API endpoint and retrieve the list of posts. Once we receive the response from the API, we loop through the posts and create HTML elements to display the title, excerpt, and read more links for each post. Finally, we append these elements to a container element on the webpage with the ID “posts-container”.
This is just one example of using the WordPress REST API to display posts on an external website. With it, you can also create, update, and delete posts, retrieve user data, manage comments, and much more.
Here are some examples of how you can use the WordPress REST API to enhance your website:
- Displaying posts: You can use it to fetch and display posts from your wordpress website on an external website or application.
- Creating custom applications: You can use it to build custom applications that interact with wordpress data, such as mobile apps, desktop apps, and web applications.
- Integrating with external services: You can use it to integrate your WordPress website with external services, such as social media platforms, payment gateways, and email marketing tools.
You can check out https://reactblog.phptutorialpoints.in/ react-based blog which fetches blog posts using WordPress REST API.
Reference :
I hope this article helps!