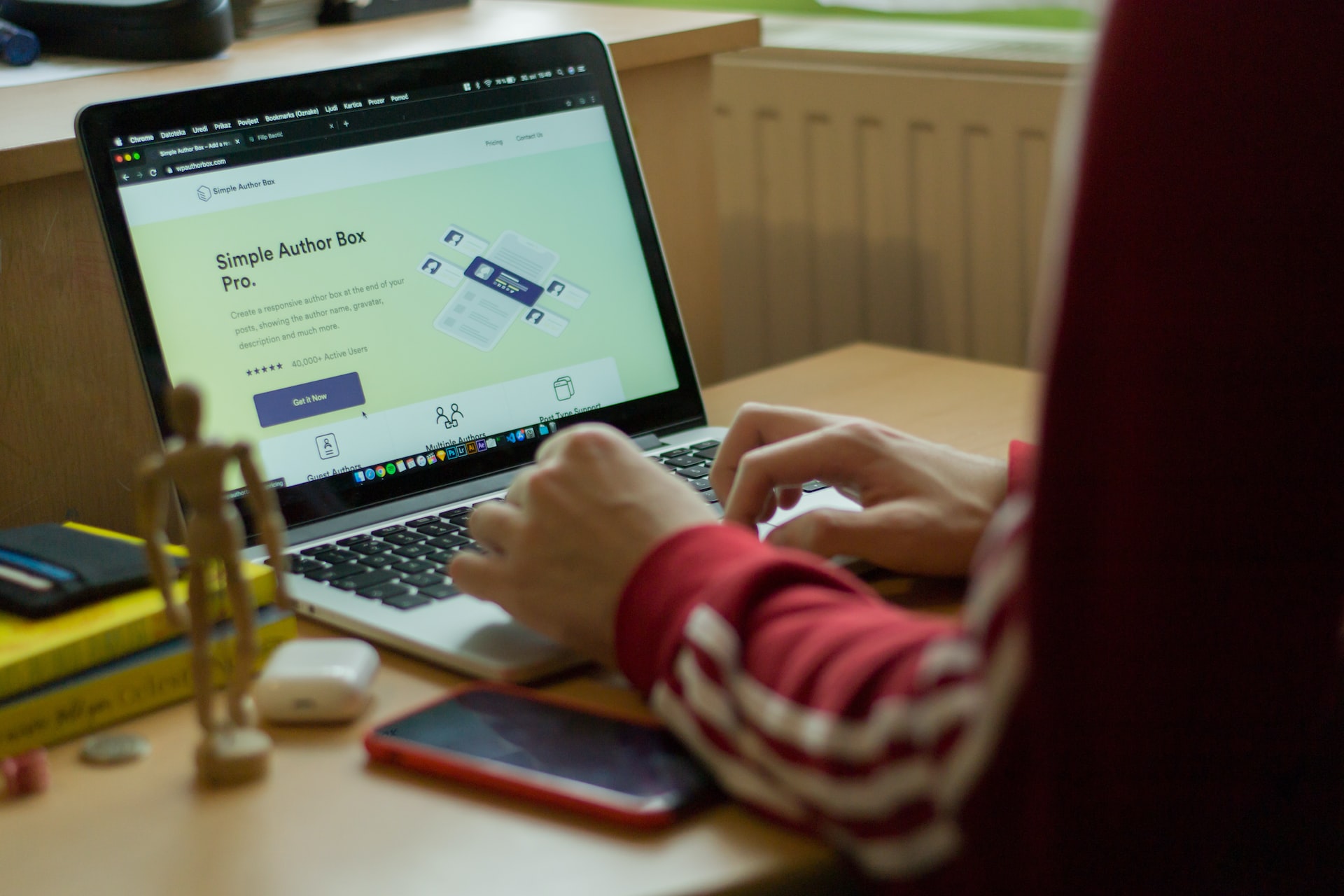
WordPress Theme Development Guide
In this article, we’ll see WordPress Theme Development Guide. You need to follow steps and recommendations to build better themes.
WordPress, the world’s most popular content management system, empowers millions of websites with its flexibility and user-friendly interface. If you’re looking to create a unique online presence, WordPress theme development offers you the opportunity to unleash your creativity and build a website tailored to your vision.
In this blog post, we’ll dive into the exciting world of WordPress theme development and explore how you can bring your ideas to life while enjoying the process.
WordPress theme development opens up a world of possibilities for customization. Unlike pre-made themes, developing your own theme allows you to have full control over the design, layout, and functionality of your website.
WordPress themes are primarily built using a combination of HTML, CSS, and PHP. If you’re already familiar with these languages, you’ll find it relatively straightforward to get started with WordPress theme development.
WordPress Theme Development Process
1). Creating a Theme using Starter Theme and Naming Theme: To create a new theme, we can use the startup themes like https://underscores.me/. Use the theme name as the project name. For theme slug, use a dash(-) when separating words instead of spaces or underscore. Theme author and URI should be the client website links and names. see below sample example for defining the theme:
/*! Theme Name: PHP Tutorial Points Theme URI: http://underscores.me/ Author: Umang Prajapati Author URI: https://phptutorialpoints.in/ Description: Description Version: 1.0.0 Tested up to: 5.4 Requires PHP: 5.6 License: GNU General Public License v2 or later License URI: LICENSE Text Domain: php-tutorial-points Tags: custom-background, custom-logo, custom-menu, featured-images, threaded-comments, translation-ready This theme, like WordPress, is licensed under the GPL. Use it to make something cool, have fun, and share what you've learned. PHP Tutorial Points is based on Underscores https://underscores.me/, (C) 2012-2020 Automattic, Inc. Underscores is distributed under the terms of the GNU GPL v2 or later. Normalizing styles have been helped along thanks to the fine work of Nicolas Gallagher and Jonathan Neal https://necolas.github.io/normalize.css/ */
By following above steps, theme is created which is first step of WordPress Theme Development
2). Basic and Useful Plugins for development: Once the theme is installed, install some essential plugins for theme development. The followings are some of the basic plugins you can use for starting theme development based on your requirements:
- https://wordpress.org/plugins/custom-post-type-ui/ (To create custom post type)
- https://www.advancedcustomfields.com/pro/ (Paid Plugin) or https://carbonfields.net/ (Free Plugin) (To create a custom field)
- https://wordpress.org/plugins/contact-form-7/ (To create a contact form)
- https://wordpress.org/plugins/contact-form-cfdb7/ (To save contact form plugin leads)
- https://wordpress.org/plugins/simple-custom-post-order/ (To reorder post/taxonomy from backend)
3). Theme Options: For managing header, footer, and contact content like logo, social media link, etc. we need to use the theme option. For a free solution, we can use the “Carbon Field” plugin-based theme option. you can learn more about it here: https://carbonfields.net/docs/containers-theme-options/.
For paid solution, we can use the Advanced Custom Fields Pro plugin. You need to define the theme options area by adding the following code in the functions.php file of the active theme. Once you add the following code, you will see the Theme Options menu on the backend. Now, you can create a field group using Advanced Custom Field Pro and add create fields like logo upload file, copyright text on theme option based on project
/* Theme Options Settings */ if( function_exists('acf_add_options_page') ) { acf_add_options_page(array( 'page_title' => 'Theme Options', 'menu_title' => 'Theme Options', 'menu_slug' => 'theme-options', 'parent_slug' => '', 'capability' => 'edit_posts', 'position' => false, 'icon_url' => false, 'redirect' => true )); acf_add_options_sub_page(array( 'page_title' => 'Theme Header Settings', 'menu_title' => 'Header Settings', 'menu_slug' => 'theme-options-header', 'capability' => 'edit_posts', 'parent_slug' => 'theme-options', 'position' => false, 'icon_url' => false )); acf_add_options_sub_page(array( 'page_title' => 'Theme Footer Settings', 'menu_title' => 'Footer Settings', 'menu_slug' => 'theme-options-footer', 'capability' => 'edit_posts', 'parent_slug' => 'theme-options', 'position' => false, 'icon_url' => false )); } /* End Theme Options Settings */
5). Including CSS & JavaScript: Use WordPress wp_enqueue_script() or wp_enqueue_style() functions to include CSS and js in theme. Rather than loading the CSS or js in your header.php file, you should load it using wp_enqueue_style or wp_enqueue_script. In order to load your CSS or js, you can enqueue it in the theme’s functions.php For example, see below how CSS is included:
wp_enqueue_style( 'slider-css', get_stylesheet_directory_uri() . '/css/slider.css',false,'1.1','all');
6). Prefixing (Prevent Conflicts): To prevent conflicts, all functions, classes, and global variables created by your theme should be prefixed. This is important because it’s impossible to know what other code is running on your user’s website. Prefixing prevents name clashes and fatal errors.
7) Theme Debugging: Always enable WordPress to debug by set “define(‘WP_DEBUG’, true);” in wp-config.php when you are developing the theme to check and fix theme-related errors. Once everything is finished with the development of the theme, then you can disable debug.
8). Use Localization: Enabling localization is helpful for translation into multiple languages. All we need to do is make sure that all strings are passed through a ‘localization function’ rather than being output directly.
Instead of this:
<?php echo 'Previous Post'; ?>
We do this instead:
<?php echo __( 'Previous Post', 'my-custom-theme' ); ?>
__() is a localization function that accepts a string and a text-domain. The function returns a translation of the string provided, or the original string if a translation isn’t available.
9). HTML and CSS validation: run your theme through HTML and CSS validation once it is finished. You can use http://validator.w3.org/ for HTML validation and http://jigsaw.w3.org/css-validator/ for CSS validation. This is necessary for WordPress Theme Development.
10). Queries: WordPress offers the possibility of fetching any kind of post from the database. There are three ways to fetch posts. But, it is good to use the WP_Query method for fetching posts rather than using the other two methods like get_posts() or query_posts() functions.
11). Escaping and Sanitizing Data: It is better to escaping and sanitizing data to protect your users from potential exploits. Escaping is the process of checking data is safe before it’s output and sanitizing is checking data before it’s saved to the database.
Escape:
WordPress has helper functions that you can use to escape data so you don’t need to build those yourself. esc_html is one example of an escaping function. This is what an unescaped output looks like:
echo get_theme_mod( 'error_page_title' );
To escape the output we do this:
echo esc_html( get_theme_mod( 'error_page_title' ) );
Some other escaping functions you should be aware of are esc_attr(), absint(), esc_url().
It’s also possible to translate and escape a string using a single function:
echo esc_html( __( '404 Not Found', 'my-custom-theme' ) );
Becomes:
echo esc_html__( '404 Not Found', 'my-custom-theme' );
// or
esc_html_e( '404 Not Found', 'my-custom-theme' );
Sanitizing
Sanitization is the process of cleaning or filtering your input data.
Let’s say we have an input field named title.
<input id=”title” type=”text” name=”title”>
You can sanitize the input data with the sanitize_text_field() function:
$title = sanitize_text_field( $_POST[‘title’] );
update_post_meta( $post->ID, ‘title’, $title );
An official list of sanitization and escaping functions can be seen here: Data Sanitization/Escaping
12) Minify Theme Assets: Always use the minified version of third-party library CSS and js files like bootstrap, owl-carousel, etc. You can also use online tools like CSS Minifier, JavaScript Minifier, CSS Compressor, JSCompress, Minify, etc.
13) Generate Screenshot from URL: You can generate theme screenshot from URL like https://s0.wp.com/mshots/v1/https://phptutorialpoints.in/
Above steps will help you in WordPress Theme Development.
Starter Themes for WordPress Theme Development:
- https://underscores.me/
- https://roots.io/sage/
- https://labs.tonik.pl/theme/
- https://understrap.com/
- https://wprig.io/
- https://barebones.dev/
- https://github.com/Themekraft/_tk
- http://gantry.org/
- https://www.getbeans.io/
- https://wordpress.org/themes/responsive/
- https://github.com/Alecaddd/awps
- https://ignition.press/
- https://upstatement.com/timber/
References:
- https://www.toptal.com/wordpress/guide-optimizing-wordpress-performance
- https://developer.wordpress.com/themes/
- https://www.dreamhost.com/wordpress/guide-to-developing-a-wp-theme/
- https://websitesetup.org/wordpress-theme-development/
- https://www.php-fig.org/psr/
- https://make.wordpress.org/core/handbook/best-practices/coding-standards/php/
- https://www.taniarascia.com/developing-a-wordpress-theme-from-scratch/
- https://www.smashingmagazine.com/2012/07/guide-wordpress-coding-standards/
- https://www.elegantthemes.com/blog/resources/a-newbies-guide-to-wordpress-coding-standards
Useful Tools:
https://www.git-tower.com/windows
WordPress theme development is a rewarding journey that allows you to shape your online presence with creativity and innovation. Embrace the power of customization, learn the essential languages and APIs, leverage starter themes and frameworks, and tap into the WordPress community for support and inspiration.
With WordPress theme development, you have the opportunity to build a website that stands out and leaves a lasting impression. So, roll up your sleeves, let your imagination soar, and embark on a friendly and fun-filled adventure in WordPress theme development. I hope this article helps you build a theme with the help of the WordPress Theme Development Guide!